介绍
在web开发中,安全是特别重要的!一般我们实现安全的手段有过滤器、拦截器…
我们使用SpringSecurity、shiro两个框架是为了更加简洁的实现安全。
Spring Security 是一个功能强大且高度可定制的身份验证和访问控制框架。 它是保护基于 Spring 的应用程序的事实标准。
Spring Security 是一个专注于为 Java 应用程序提供身份验证和授权的框架。 与所有 Spring 项目一样,Spring Security 的真正强大之处在于它可以轻松扩展以满足自定义需求
注意点
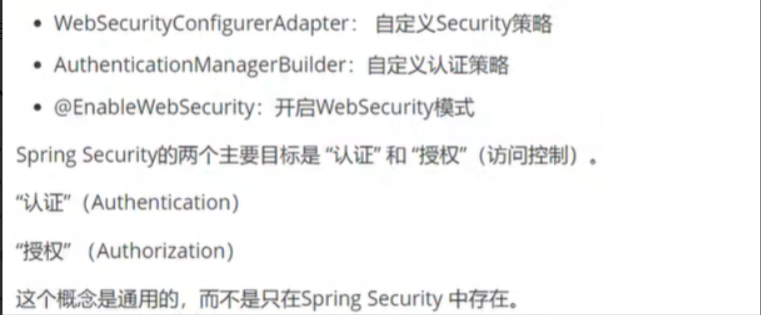
相关依赖
1 2 3 4 5 6 7 8 9 10 11 12 13
| //SpringSecurity配置依赖 <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
<dependency> <groupId>org.thymeleaf.extras</groupId> <artifactId>thymeleaf-extras-springsecurity5</artifactId> </dependency>
|
配置实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| package com.demo.config; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfiguration; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@EnableWebSecurity public class securityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { } @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { }
}
|
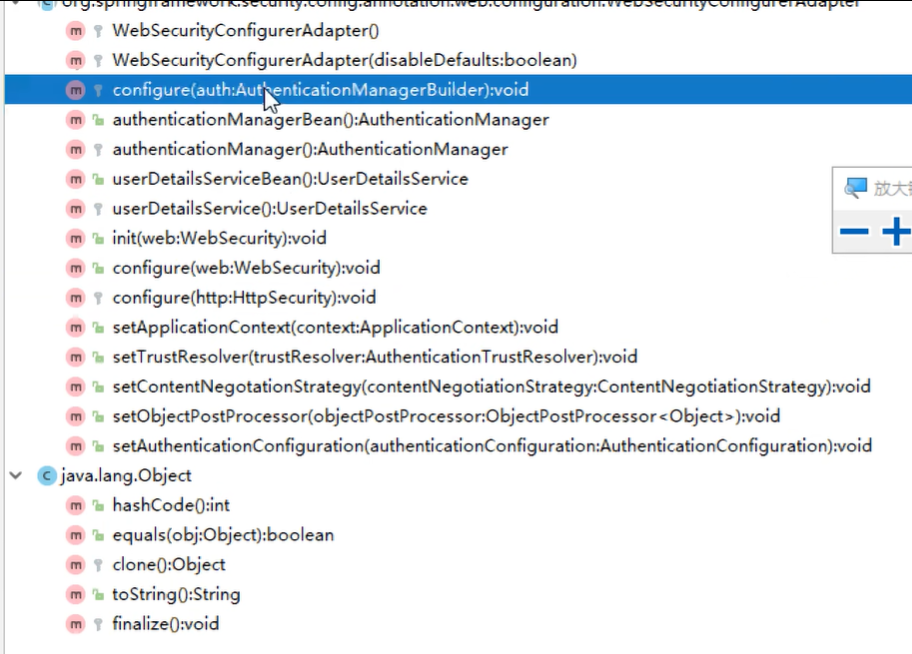
最主要的两个方法
认证
通过实现对相关用户的权限赋值,从而实现不同权限展现出来的东西也不同
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication().passwordEncoder(new BCryptPasswordEncoder()) .withUser("root").password(new BCryptPasswordEncoder().encode("520010")).roles("vip1","vip2") .and() .withUser("tempUser").password(new BCryptPasswordEncoder().encode("520010")).roles("vip1") .and() .withUser("rayce").password(new BCryptPasswordEncoder().encode("520010")).roles("vip1","vip2","vip3");
} }
|
授权
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| @Override protected void configure(HttpSecurity http) throws Exception { http.authorizeRequests() .antMatchers("/").permitAll() .antMatchers("/first1/**").hasRole("vip1") .antMatchers("/first2/**").hasRole("vip2") .antMatchers("/first3/**").hasRole("vip3");
http.formLogin().loginPage("/to/login").usernameParameter("username").passwordParameter("password").loginProcessingUrl("/login");
http.csrf().disable(); http.rememberMe().rememberMeParameter("remember");
http.logout().logoutSuccessUrl("/index"); }
|
前端页面使用security
注意修改pom中的parent版本为3.0.4.RELESE,这样才会显示我们需要展示的效果,但是页面效果会大打折扣
添加命名空间
命名空间改为xmlns:sec=”http://www.thymeleaf.org/extras/spring-security5“

使用:
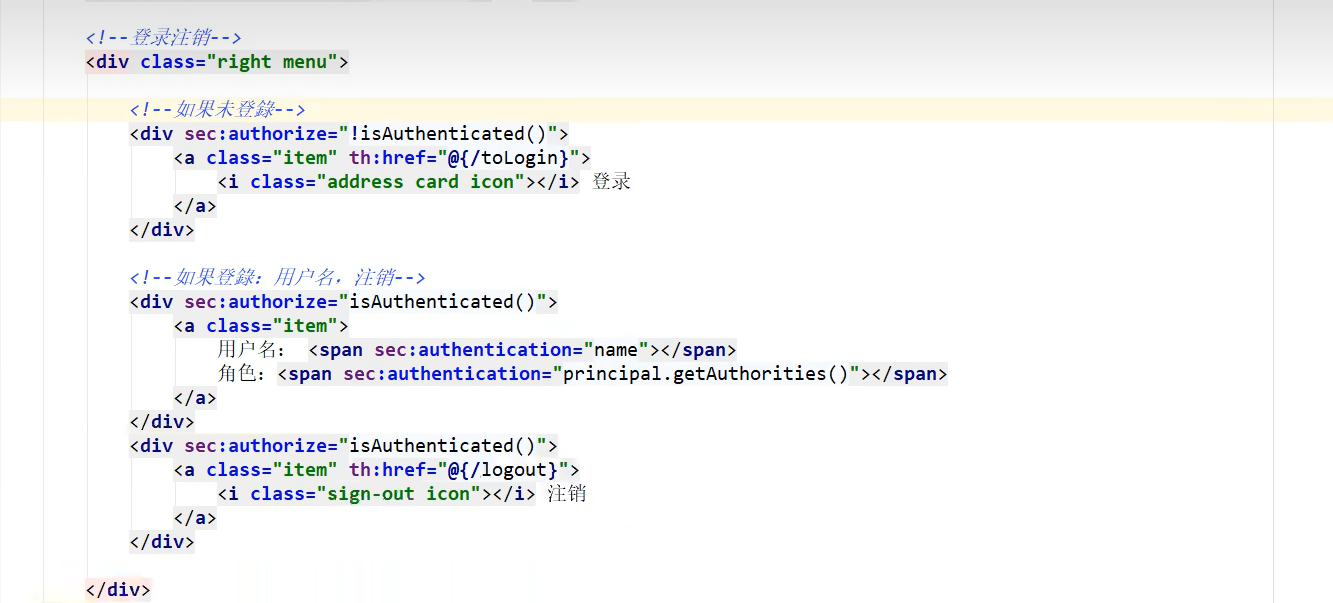
对于不同板块不同权限的人可见的设置
1 2 3 4 5 6 7 8 9
| <div class="XXX1" sec:authorize="hasRoles('vip1')"> //需要展示的内容 </div> <div class="XXX2" sec:authorize="hasRoles('vip2')"> //需要展示的内容 </div> <div class="XXX3" sec:authorize="hasRoles('vip3')"> //需要展示的内容 </div>
|